Components
Image
The Image component is used to display images.
Usage
The Image component is used to display images. with capability can load from anything, like url, colors, bitmap, drawable or etc. Engine for image processing we use Glide
Basic
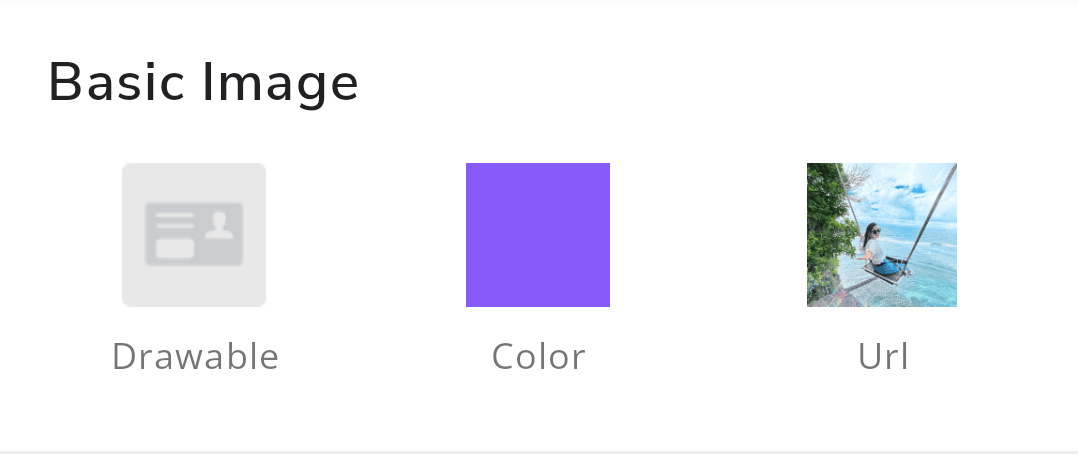
LgnImageView supports loading images via xml including:
Drawable
Static in xml
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"android:src="@drawable/img_ktp"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent" />
Dynamic using Kotlin*
...with(binding) {containerBase.addView( //ViewGroup for Dynamic LayoutLgnImageView(requiredContext()).apply {resId = R.drawable.img_ktp // Using ResId//Or Using Instance of DrawableresDrawable = ContextCompat.getDrawable(requireContext(), R.drawable.img_ktp)//Your View's customization here
Colors
Static in xml
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"android:src="?attr/colorPrimary"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent" />
Dynamic using Kotlin*
...with(binding) {containerBase.addView( //ViewGroup for Dynamic LayoutLgnImageView(requiredContext()).apply {colors = ContextCompat.getColor(requireContext(), R.color.colorPrimary)//Your View's customization here},LinearLayout.LayoutParams( //For example we use viewgroup LinearLayout
Image Url
Static in xml
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"app:layout_constraintTop_toTopOf="parent" />
Dynamic using Kotlin*
...with(binding) {containerBase.addView( //ViewGroup for Dynamic LayoutLgnImageView(requiredContext()).apply {url = "https://oratakashi.com/images/weeding/female.jpg"//Your View's customization here},LinearLayout.LayoutParams( //For example we use viewgroup LinearLayout
Image Radius
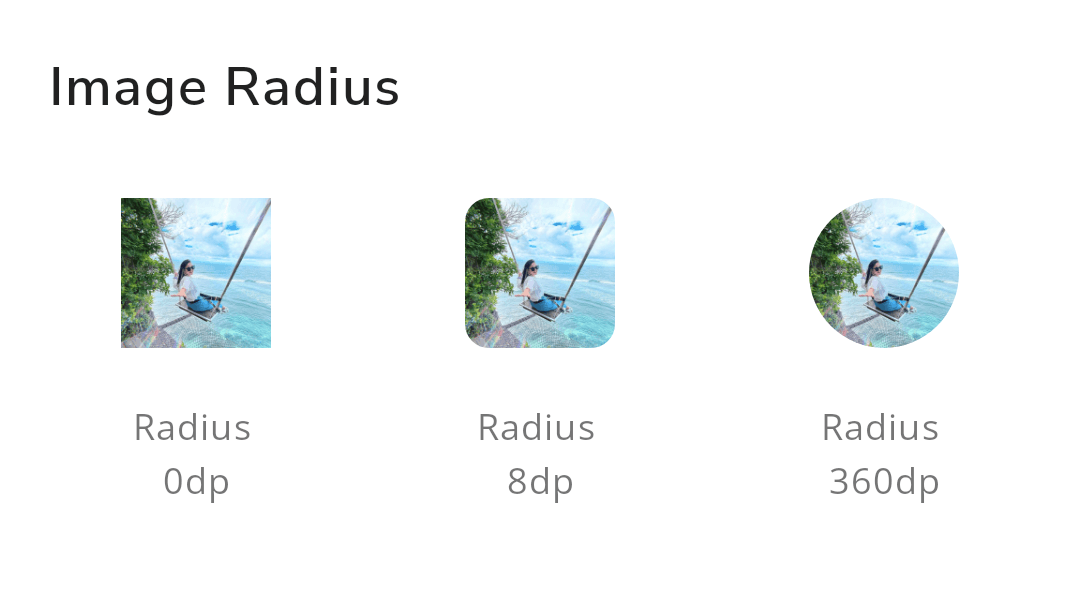
LgnImageView supports images radius via xml including:
Rectangle
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"app:src="https://oratakashi.com/images/weeding/female.jpg"android:radius="@dimen/dimen_0dp"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"
Rounded
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"app:src="https://oratakashi.com/images/weeding/female.jpg"android:radius="@dimen/dimen_8dp"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"
Circle
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"android:layout_marginBottom="@dimen/dimen_8dp"app:src="https://oratakashi.com/images/weeding/female.jpg"android:radius="@dimen/dimen_360dp"app:layout_constraintBottom_toTopOf="@+id/textView"app:layout_constraintEnd_toEndOf="parent"app:layout_constraintStart_toStartOf="parent"
Image Caching
LgnImageView can using cache by default, LgnImageView checks multiple layers of caches before starting a new request for an image:
Active resources - Is this image displayed in another View right now?
Memory cache - Was this image recently loaded and still in memory?
Resource - Has this image been decoded, transformed, and written to the disk cache before?
Data - Was the data this image was obtained from written to the disk cache before?
The first two steps check to see if the resource is in memory and if so, return the image immediately. The second two steps check to see if the image is on disk and return quickly, but asynchronously.
You can set of available caching strategies for media:
ALL
Caches remote data with both DATA and RESOURCE, and local data with RESOURCE only.
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:cache="ALL" />
AUTOMATIC
Tries to intelligently choose a strategy based on the data source of the DataFetcher and the EncodeStrategy of the ResourceEncoder (if an ResourceEncoder is available).
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:cache="AUTOMATIC" />
DATA
Writes retrieved data directly to the disk cache before it’s decoded.
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:cache="DATA" />
NONE
Saves no data to cache. this is default configuration if you not set cache.
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:cache="NONE" />
RESOURCE
Writes resources to disk after they’ve been decoded.
<com.telkom.legion.component.image.LgnImageViewandroid:layout_width="50dp"android:layout_height="50dp"app:src="https://oratakashi.com/images/weeding/female.jpg"app:cache="RESOURCE" />
Attributes
Attribute Name | Xml Attrs | Related method(s) | Description |
---|---|---|---|
Load Drawable Image | app:src or android:src | resDrawable or resId | To load drawable image from xml |
Load Colors | app:src or android:src | colors | To load colors in image from xml |
Load Image Url | app:src | url | To load Url in image from xml |
Set Corner Radius | android:radius | radius | To set image radius in image from xml |
Set Cache | app:cache | cache | To set cache in image from xml |
References
Do you have feedback?
Please let us know to make it better