Components
Avatar
A graphical representation of an object or entity
Usage
Avatar are used to defines graphical representation of a user or the user’s character or persona
Use Legion avatar styles to modify avatar with lots of attributes that make you easier
import LegionUI
Variant
Legion iOS Have 3 Variant of Avatar :
Avatar Image
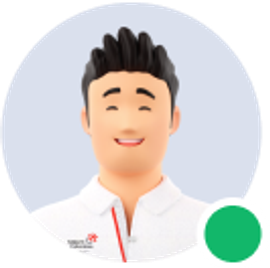
A. Avatar Image Local
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",).setBadgeOn(isOn: true)}
B. Avatar Image System
var body: some View {LGNAvatarImage(systemName: "person.3.fill",).setBadgeOn(isOn: true)}
C. Avatar Image Async
var body: some View {LGNAvatarImage(urlString: "https://i.ibb.co/1btpjhH/persegipanjangvertical.jpg",).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)}
Avatar Icon
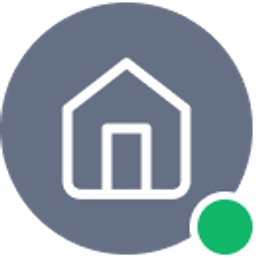
A. Avatar Icon Local
var body: some View {LGNAvatarIcon(imageNamed: "SomeAssetName",).setBadgeOn(isOn: true)}
B. Avatar Icon System
var body: some View {LGNAvatarIcon(systemName: "house").setBadgeOn(isOn: true)}
C. Avatar Icon Async
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)}
Avatar Initial

Avatar Initial should add label: "value"
where value
consist the avatar initial
var body: some View {LGNAvatarInitials(label: "Telkom Indonesia",).setBadgeOn(isOn: true)}
Attribute
Legion Have 3 Attributes for Costumize Avatar :
Size
This size attribute for user to choose the size of the avatar.
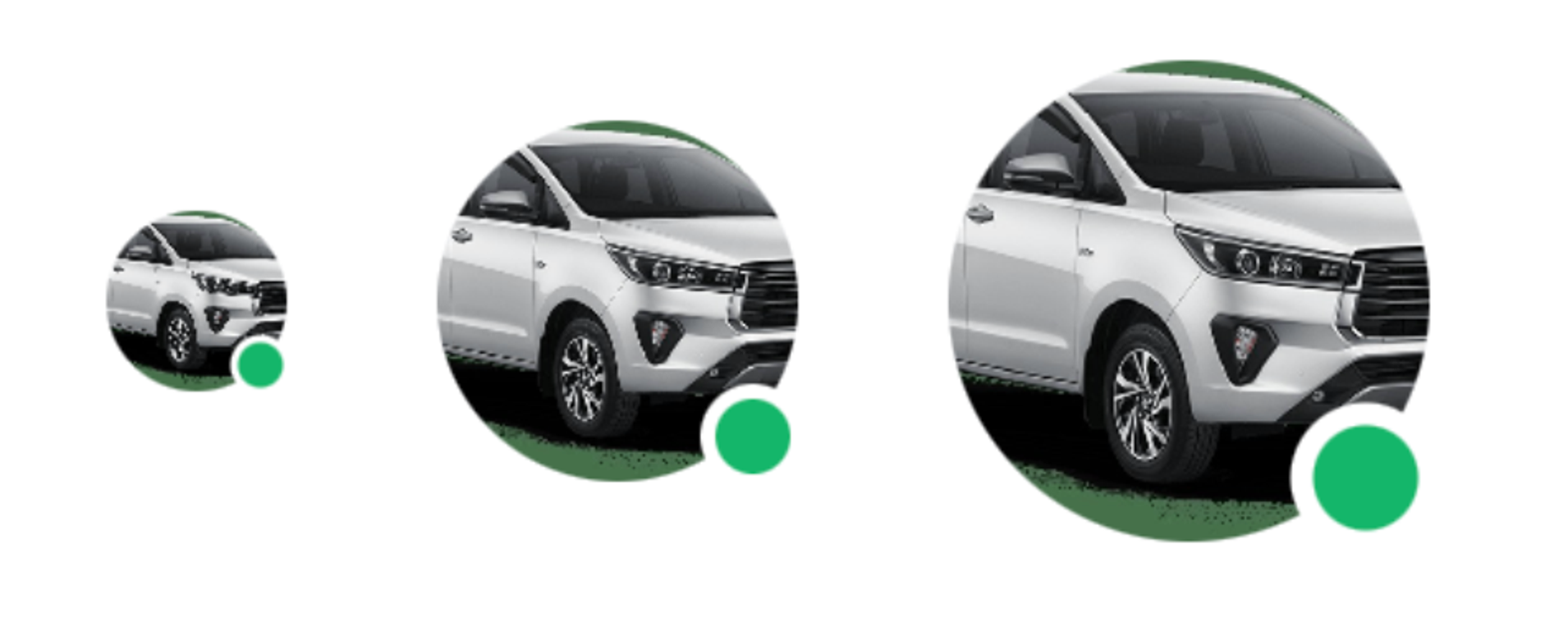
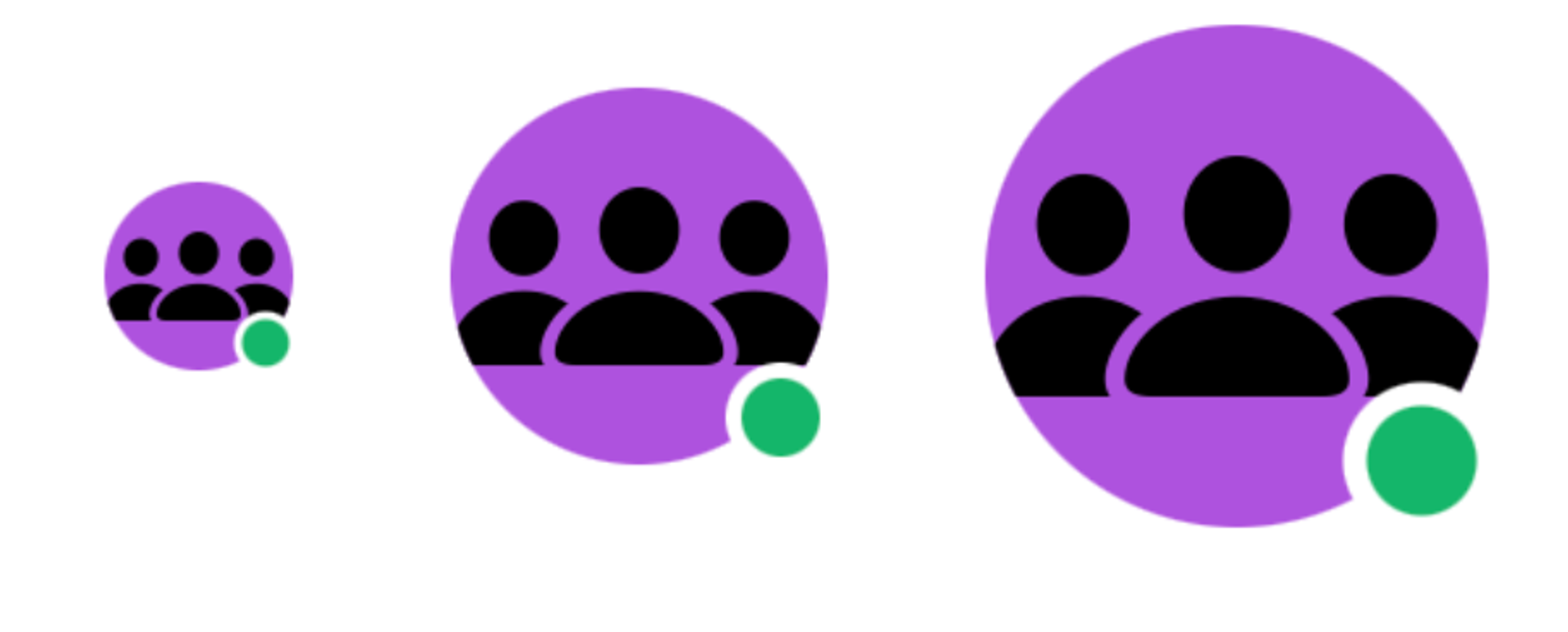
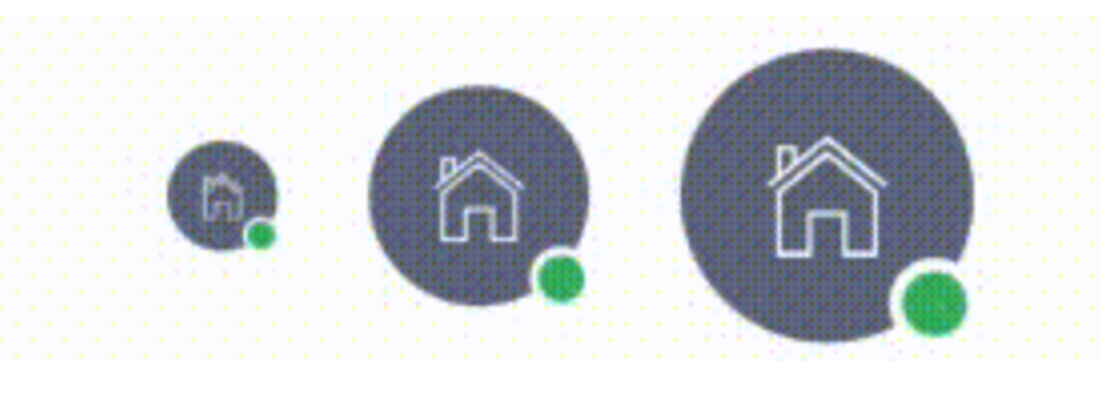
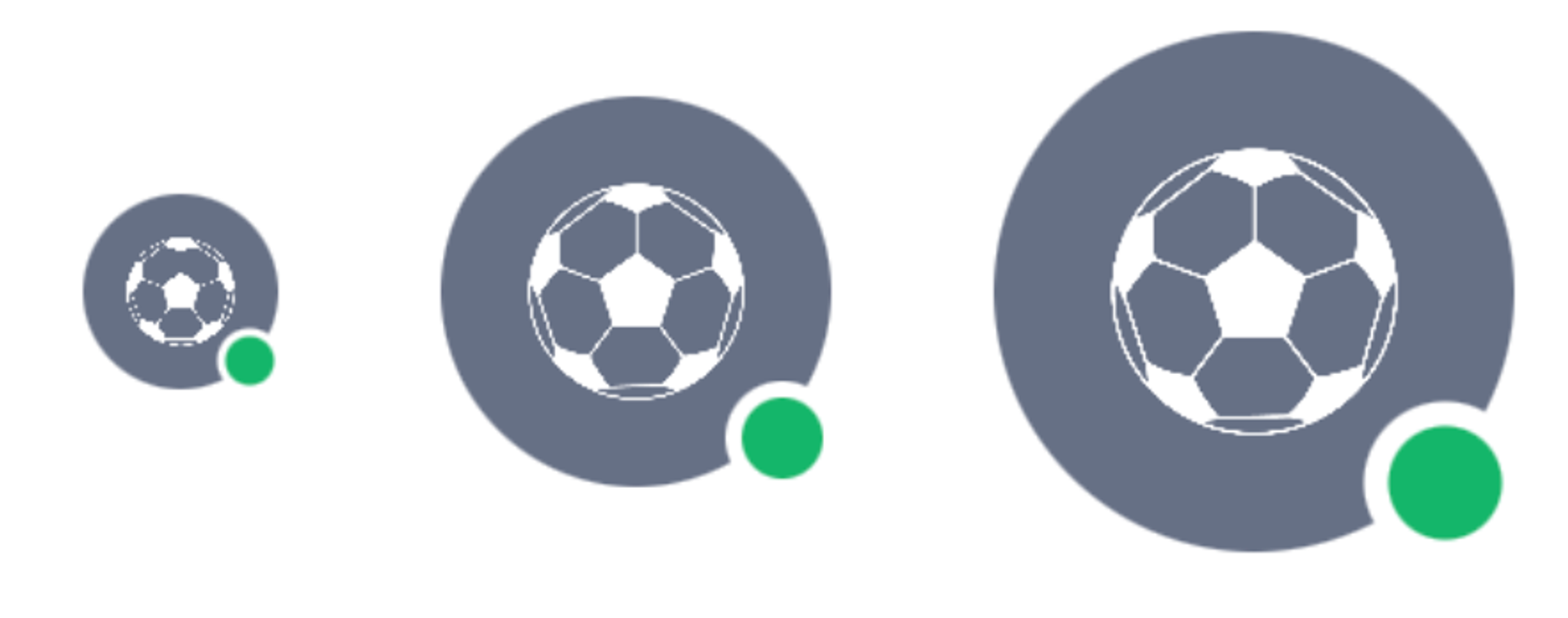
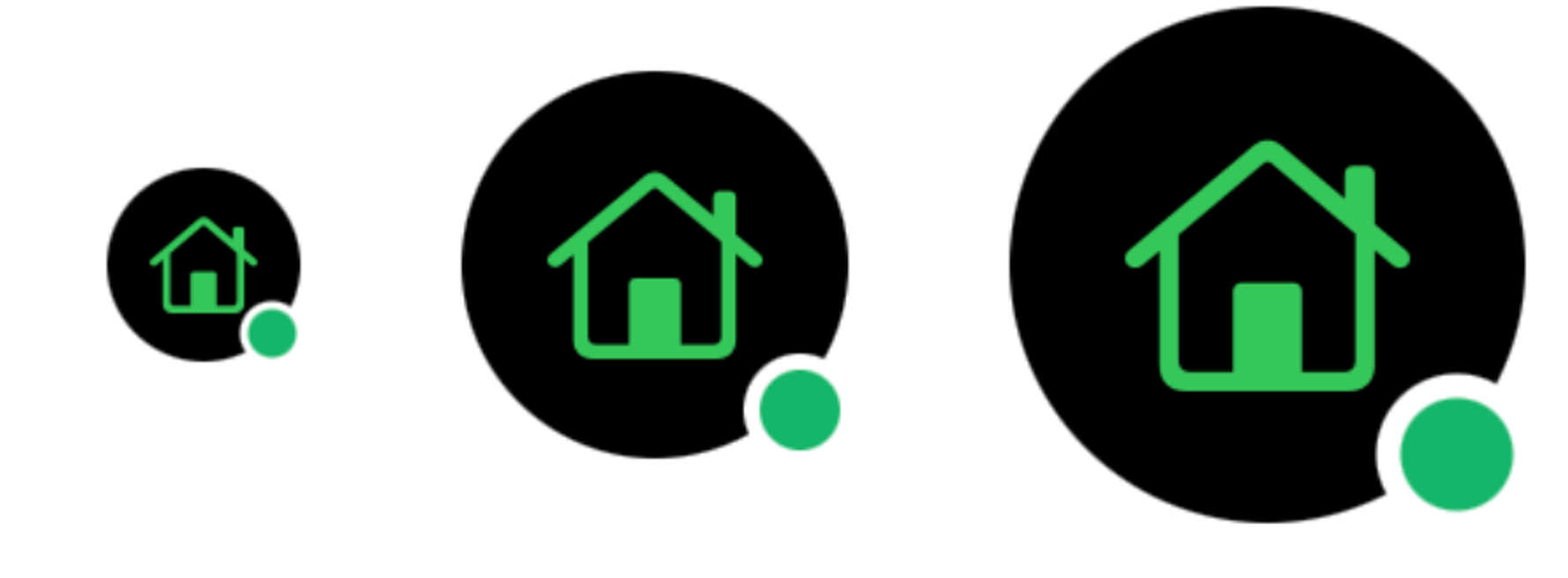
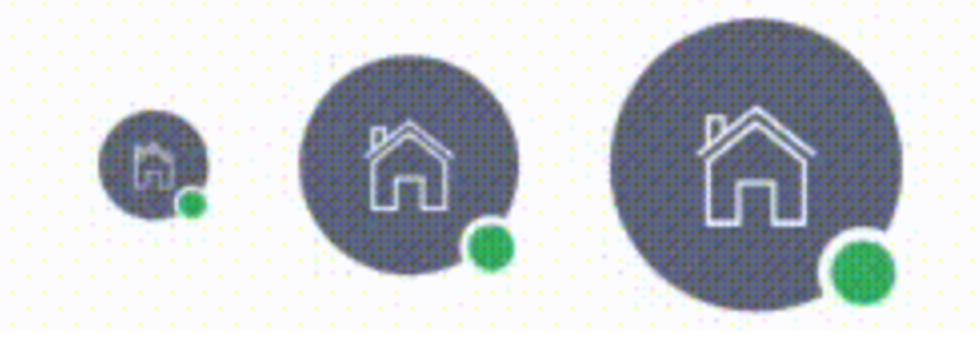
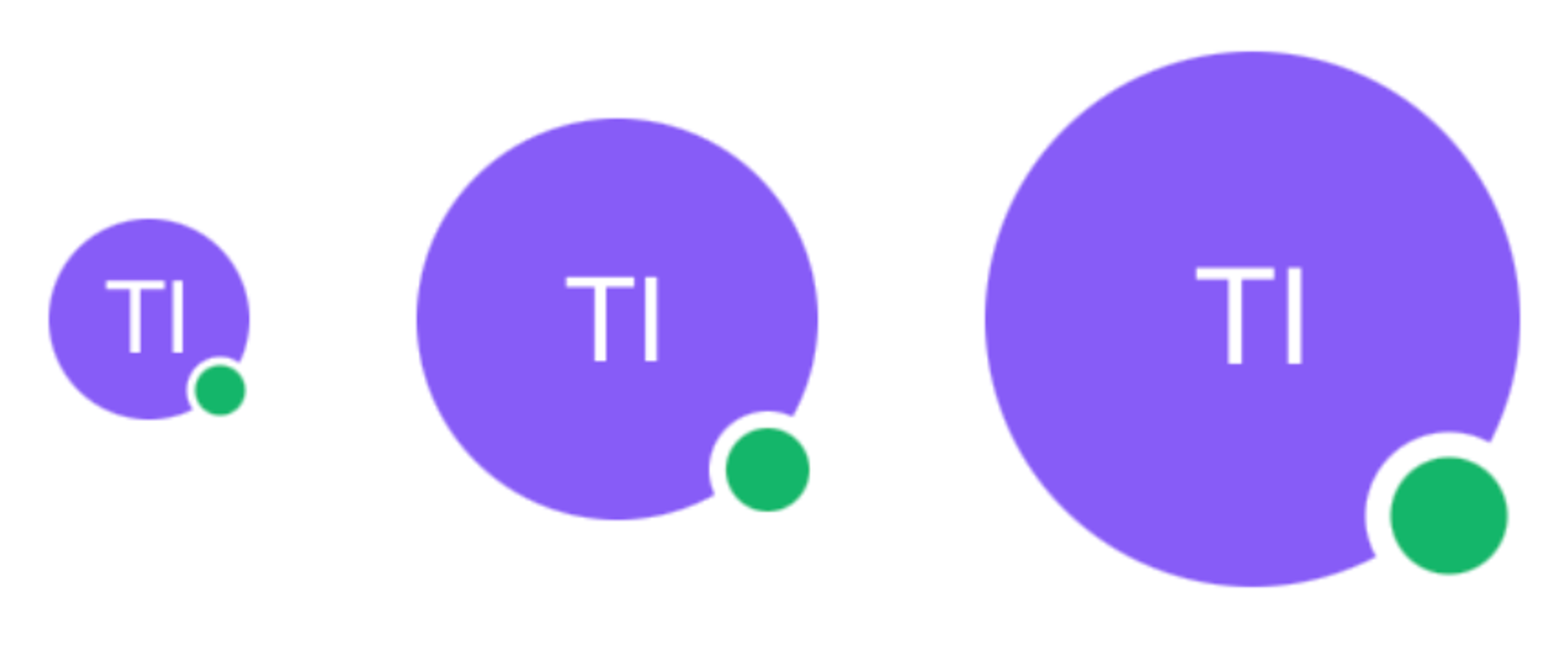
Size | Small | Medium | Large |
Choose type avatar LGNAvatarImage
, LGNAvatarIcon
or LGNAvatarInitial
, add sources imageNamed: "Link"
, systemName: "Link"
or urlString: "Link"
where Link
consist the value of the image. And add size: .sizeAvatar
where .sizeAvatar
consist .small
, .medium
or .large
This code sample demonstrates how to modify the size of the avatar :
A. Avatar Image Local Small
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .small).setBadgeOn(isOn: true)}
B. Avatar Image System Small
var body: some View {LGNAvatarImage(systemName: "person.3.fill",size: .small).setBadgeOn(isOn: true)}
C. Avatar Image Async Small
var body: some View {LGNAvatarImage(urlString: "https://i.ibb.co/1btpjhH/persegipanjangvertical.jpg",size: .smallsize: .small).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
D. Avatar Image Local Medium
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .medium).setBadgeOn(isOn: true)}
E. Avatar Image System Medium
var body: some View {LGNAvatarImage(systemName: "person.3.fill",size: .medium).setBadgeOn(isOn: true)}
F. Avatar Image Async Medium
var body: some View {LGNAvatarImage(urlString: "https://i.ibb.co/1btpjhH/persegipanjangvertical.jpg",size: .medium).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
G. Avatar Image Local Large
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .large).setBadgeOn(isOn: true)}
H. Avatar Image System Large
var body: some View {LGNAvatarImage(systemName: "person.3.fill",size: .large).setBadgeOn(isOn: true)}
I. Avatar Image Async Large
var body: some View {LGNAvatarImage(urlString: "https://i.ibb.co/1btpjhH/persegipanjangvertical.jpg",size: .large).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
J. Avatar Icon Local Small
var body: some View {LGNAvatarIcon(imageNamed: "SomeAssetName",size: .small).setBadgeOn(isOn: true)}
K. Avatar Icon System Small
var body: some View {LGNAvatarIcon(systemName: "house",size: .small).setBadgeOn(isOn: true)}
L. Avatar Icon Async Small
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .small).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
M. Avatar Icon Local Medium
var body: some View {LGNAvatarIcon(imageNamed: "SomeAssetName",size: .medium).setBadgeOn(isOn: true)}
N. Avatar Icon System Medium
var body: some View {LGNAvatarIcon(systemName: "house",size: .medium).setBadgeOn(isOn: true)}
O. Avatar Icon Async Medium
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .medium).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
P. Avatar Icon Local Large
var body: some View {LGNAvatarIcon(imageNamed: "SomeAssetName",size: .large).setBadgeOn(isOn: true)}
Q. Avatar Icon System Large
var body: some View {LGNAvatarIcon(systemName: "house",size: .large).setBadgeOn(isOn: true)}
R. Avatar Icon Async Large
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .large).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
S. Avatar Initial Small
var body: some View {LGNAvatarInitials(label: "Telkom Indonesia",size: .small).setBadgeOn(isOn: true)}
T. Avatar Initial Medium
var body: some View {LGNAvatarInitials(label: "Telkom Indonesia",size: .medium).setBadgeOn(isOn: true)}
U. Avatar Intial Large
var body: some View {LGNAvatarInitials(label: "Telkom Indonesia",size: .large).setBadgeOn(isOn: true)}
Ratio


This avatar attribute for user to choose the ration image position inside the avatar.
Choose size of the avatar size: .sizeAvatar
where .sizeAvatar
consist .small
, .medium
or .large
and Use this function .aspectRatio(contentMode: .ratio)
where ratio
consist fit
or fill
if you add fit
must add the backgroundColor: .color
below size: .sizeAvatar
where color
is the color value
This code sample demonstrates how to modify the ratio of the avatar :
A. Small Fit
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .small,backgroundColor: .purple).setBadgeOn(isOn: true).aspectRatio(contentMode: .fit)}
B. Small Fill
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .small).setBadgeOn(isOn: true).aspectRatio(contentMode: .fill)}
C. Medium Fit
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .mediumbackgroundColor: .purple).setBadgeOn(isOn: true).aspectRatio(contentMode: .fit)}
D. Medium Fill
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .medium).setBadgeOn(isOn: true).aspectRatio(contentMode: .fill)}
E. Large Fit
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .largebackgroundColor: .purple).setBadgeOn(isOn: true).aspectRatio(contentMode: .fit)}
F. Large Fill
var body: some View {LGNAvatarImage(imageNamed: "SomeAssetName",size: .large).setBadgeOn(isOn: true).aspectRatio(contentMode: .fill)}
Show Progress

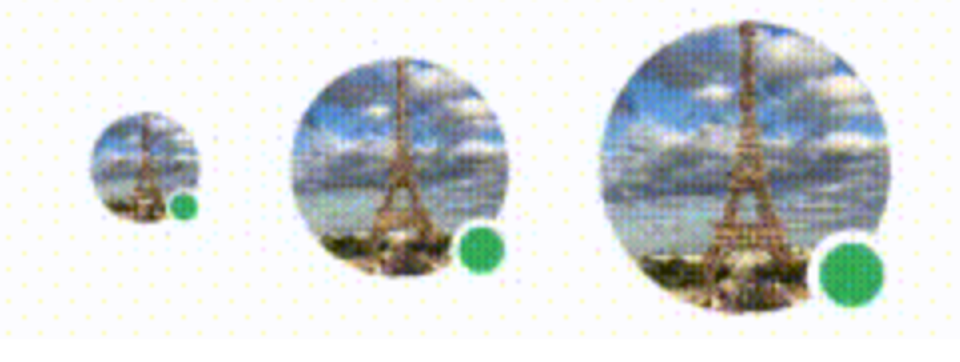
This label attribute to customize show progress async from url for avatar.
add sources imageNamed: "Link"
, systemName: "Link"
or urlString: "Link"
where Link
consist the value of the image. And add size: .sizeAvatar
where .sizeAvatar
consist .small
, .medium
or .large
finish it with .setPlaceholder(isProgressViewOn: true)
or .setPlaceholder(isProgressViewOn: false)
This code sample demonstrates how to modify the show progress of the async avatar :
A. Small Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .small).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
B. Small Not Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .small).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: false)
C. Medium Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .medium).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
D. Medium Not Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .medium).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: false)
E. Large Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .large).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: true)
F. Large Not Show Progress
var body: some View {LGNAvatarIcon(urlString: "https://i.ibb.co/yQPCHqz/houseicon.png",size: .large).setBadgeOn(isOn: true).setPlaceholder(isProgressViewOn: false)
Example Project
Avatar Image
LGNAvatarImage(urlString: "https://i.ibb.co/1btpjhH/persegipanjangvertical.jpg",size: .large,backgroundColor: .green).setBadgeOn(isOn: true).setBadgeColor(color: .purple).aspectRatio(contentMode: .fit).setPlaceholder(
Avatar Icon
LGNAvatarIcon(systemName: "house",size: .large,iconColor: .yellow,backgroundColor: .LGNTheme.success500).aspectRatio(contentMode: .fit).setBadgeOn(isOn: true).setBadgeColor(color: .red)
Avatar Initial
LGNAvatarInitials(label: "Telkom Indonesia",size: .large,fontFamily: "HelveticaNeue-MediumItalic",textColor: .blue,backgroundColor: .red).setBadgeOn(isOn: true).setBadgeColor(color: .yellow)
Properties
Properties : LGNAvatarImage
Properties | Description | Default Value |
---|---|---|
imageNamed | The name of the local asset that you want to use. | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary100 |
systemName | The name of the system name from SF Symbols that you want to use. (https://developer.apple.com/design/resources/#sf-symbols) | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary100 |
urlString | The url asset that you want to use. We recommend you to use .jpg, .jpeg, and .png format. | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary100 |
Properties: LGNAvatarIcon
Properties | Description | Default Value |
---|---|---|
imageNamed | The name of the local asset that you want to use. | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
iconColor | The color of the icon. | Color.white |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary500 |
systemName | The name of the system name from SF Symbols that you want to use. (https://developer.apple.com/design/resources/#sf-symbols ) | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
iconColor | The color of the icon. | Color.white |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary500 |
urlString | The url asset that you want to use. We recommend you to use .jpg, .jpeg, and .png format. | required or no default value |
size | The size of the avatar, there are three size: .small , .medium , .large | required or no default value |
iconColor | The color of the icon. | Color.white |
backgroundColor | The color of the avatar background. | Color.LGNTheme.tertiary500 |
Properties: LGNAvatarInitials
Properties | Description | Default Value |
---|---|---|
label | The long text that you want to take the initials of to appear on the avatar. | required or no default value |
fontFamily | The font family for the label. | "" |
textColor | The color of the text string. | Color.white |
backgroundColor | The color of the avatar background. | Color.LGNTheme.primary500 |
Do you have feedback?
Please let us know to make it better